Factorial of a number in computer science and mathematics, the factorial of a non-negative integer n
, denoted as n!
, is the product of all positive integers less than or equal to n
. It is defined as follows:
๏ฟฝ!=๏ฟฝร(๏ฟฝโ1)ร(๏ฟฝโ2)รโฆร2ร1n!=nร(nโ1)ร(nโ2)รโฆร2ร1
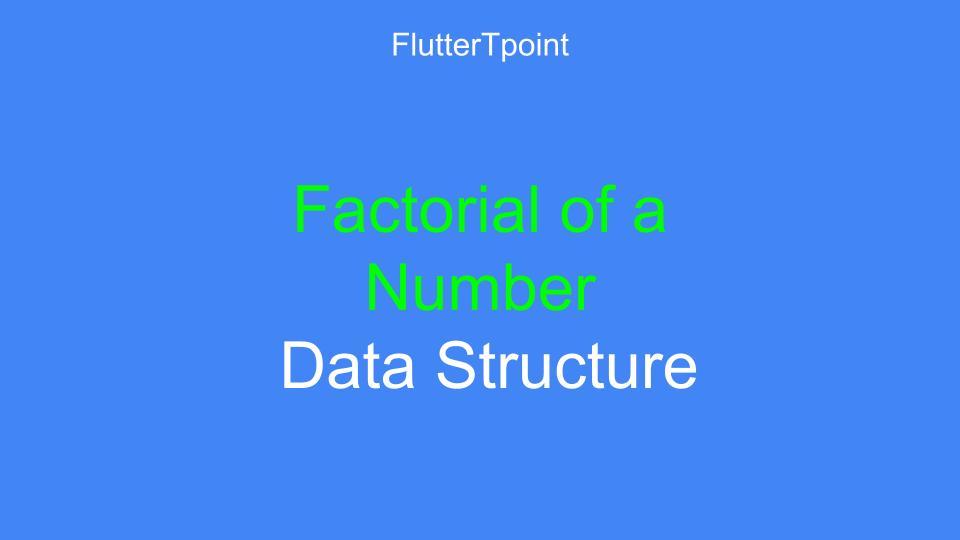
Here’s an explanation of how to calculate the factorial of a number using examples in Java, PHP, Dart, and JavaScript:
Factorial Examples of a Number
Below are some examples in different-different languages.
Java Example:
public class FactorialExample {
public static int factorial(int n) {
if (n == 0 || n == 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
public static void main(String[] args) {
int number = 5; // Change this to the desired number
int result = factorial(number);
System.out.println("Factorial of " + number + " is: " + result);
}
}
PHP Example:
<?php
function factorial($n) {
if ($n == 0 || $n == 1) {
return 1;
} else {
return $n * factorial($n - 1);
}
}
$number = 5; // Change this to the desired number
$result = factorial($number);
echo "Factorial of $number is: $result";
?>
Dart Example:
int factorial(int n) {
if (n == 0 || n == 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
void main() {
int number = 5; // Change this to the desired number
int result = factorial(number);
print("Factorial of $number is: $result");
}
JavaScript Example:
function factorial(n) {
if (n === 0 || n === 1) {
return 1;
} else {
return n * factorial(n - 1);
}
}
const number = 5; // Change this to the desired number
const result = factorial(number);
console.log(`Factorial of ${number} is: ${result}`);
Replace the number
variable with the desired integer for which you want to calculate the factorial. Since it defines the rationale for factorial computation, the recursive function factorial is used in all of the instances..
Output:
If you run any of the above code for 5 then you will get:
Factorial of 5 is: 120
Inorder Traversal in Binary Search Tree Full Explanation
Postorder Traversal in Binary Search Tree With Full Explanation
Preorder Traversal In Binary Search Tree Full Explanation
Binary Search Tree Traversal With Full Examples And Explanation
Difference Between Binary Tree and Binary Search Tree With Examples
Searching in Binary Search Tree | FlutterTPoint
FutureBuilder in Flutter With Examplesfuturebuilder flutter
Binary Search Tree With Full Examples FlutterTPoint
Thank you for reaching out the FlutterTpoint. Hardly recommended to you: